- A multidimensional array is an array of arrays.
- Each element of a multidimensional array is an array itself.
- Multidimensional array represents
- Two Dimensional Array
- Three Dimensional Array.
Two Dimensional Array:
- In Two Dimensional Array the data stored in row and columns, and we can access the record using both the row index and column index.
- Two Dimensional Array in Java is the simplest form of Multi-Dimensional Array.
- Two dimensional array code snippet.
- Data_Type[][] Array_Name = new int[Row_Size][Column_Size];
- Row_Size: Number of Row elements an array can store. For example, Row_Size = 5, then the array will have five rows.
- Column_Size: Number of Column elements an array can store. For example, Column_Size = 6, then the array will have 6 Columns.
- If you already initialized a two dimensional array in Java, then
- double [][] anStudentArray; // Declaration of Two dimensional array in java
- anStudentArray = new int[5][3];

Initialization of Two Dimensional Array in Java
Two Dimensional Array First Approach:
- Initialize Array elements more traditionally.
int[][] Student_Marks = new int[2][3];
Two dimensional Array Second Approach:
- We did not mention the column size. However, the Jcompiler is intelligent enough to calculate the size by checking the number of elements inside the column.
int[][] Student_Marks = new int[2][];
Two dimensional array Third Approach:
- We did not mention the row size and column size. However, the compiler is intelligent enough to calculate the size by checking the number of elements inside the row and column.
int[][] Employees = { {10, 20, 30}, {15, 25, 35}, {22, 44, 66}, {33, 55, 77} };
Fourth Approach for Two Dimensional array in Java:
- Array of size 5 rows * 3 columns, but we only assigned values for one row. In this situation, the remaining values assigned to default values (0 in this case).
int[][] anIntegerArray = new int[5][3];
anIntegerArray[0][0] = 10;
anIntegerArray[0][1] = 20;
anIntegerArray[0][2] = 30;
EXAMPLE FOR TWO DIMENSIONAL ARRAY:
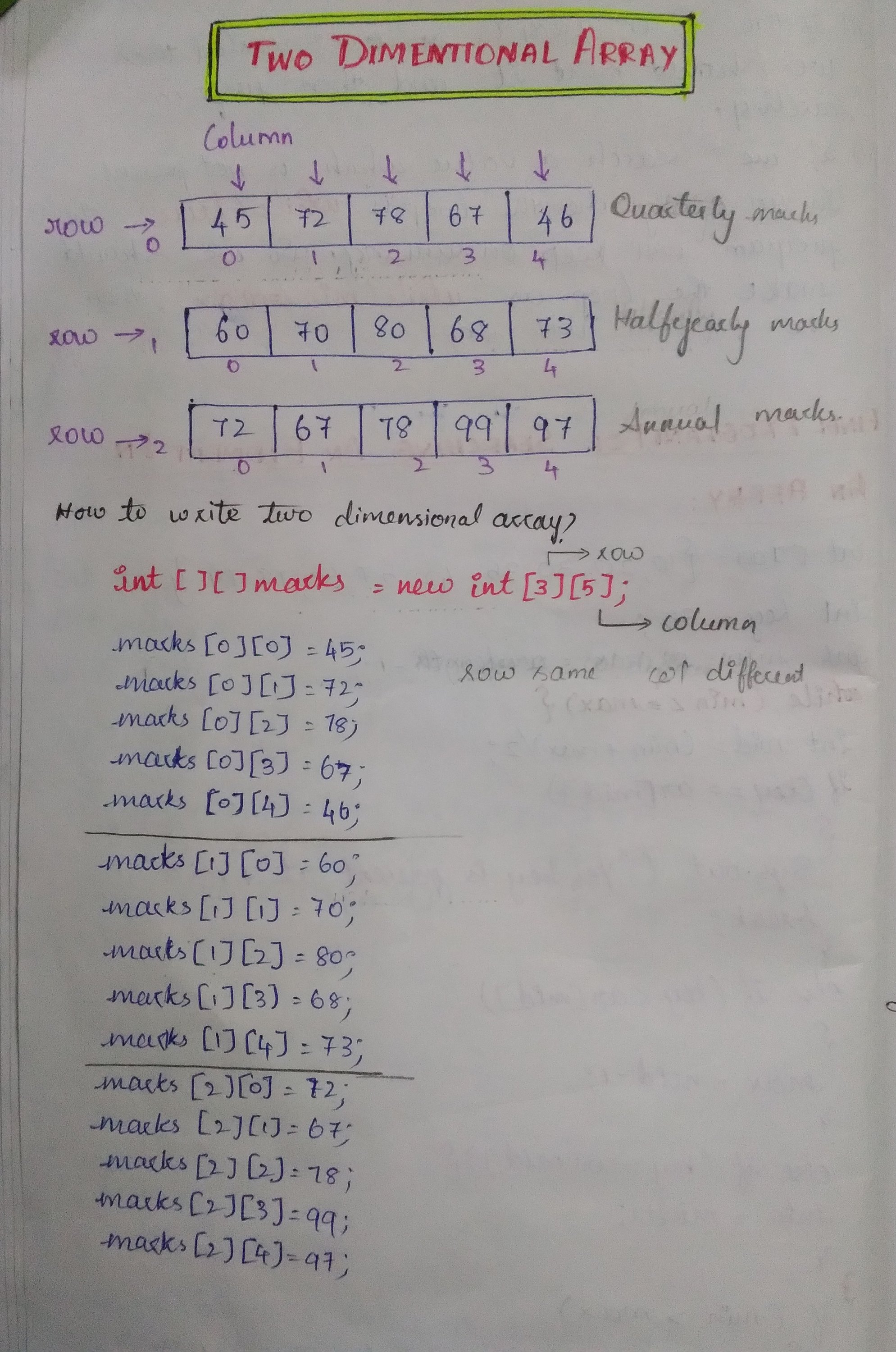
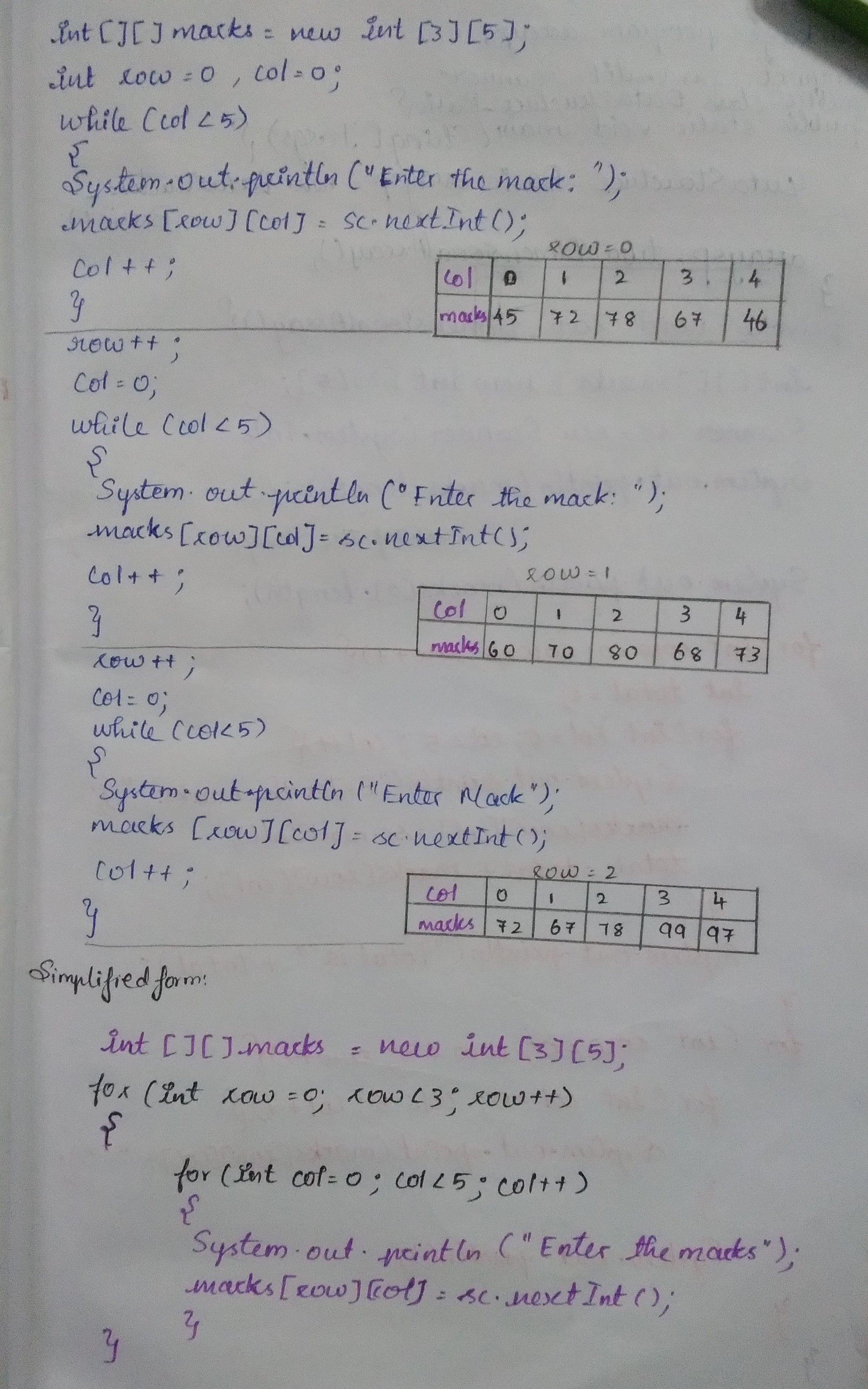
package program_arrays;
import java.util.Scanner;
public class DataStructure_Basic {
public static void main(String[] args) {
DataStructure_Basic arraysp = new DataStructure_Basic();
arraysp.two_DimensionalArray();
}
private void two_DimensionalArray() {
// TODO Auto-generated method stub
int[][] marks = new int[3][5];
Scanner sc = new Scanner(System.in);
System.out.println(marks.length);// in 2 dimensional array the length is row length
System.out.println(marks[0].length);// this shows the column length of array
for (int row = 0; row < 3; row++) {
int total = 0;
for (int col = 0; col < 5; col++) {
System.out.println("Enter the marks: ");
marks[row][col] = sc.nextInt();
total = total + marks[row][col];
}
System.out.println("Total is " + total);
}
for (int row = 0; row < 3; row++) {
for (int col = 0; col < 5; col++) {
System.out.print(marks[row][col] + " ");
}
System.out.println();
}
}
}
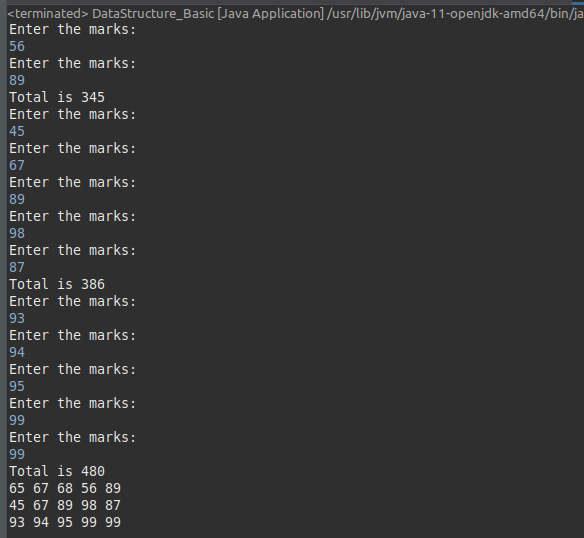
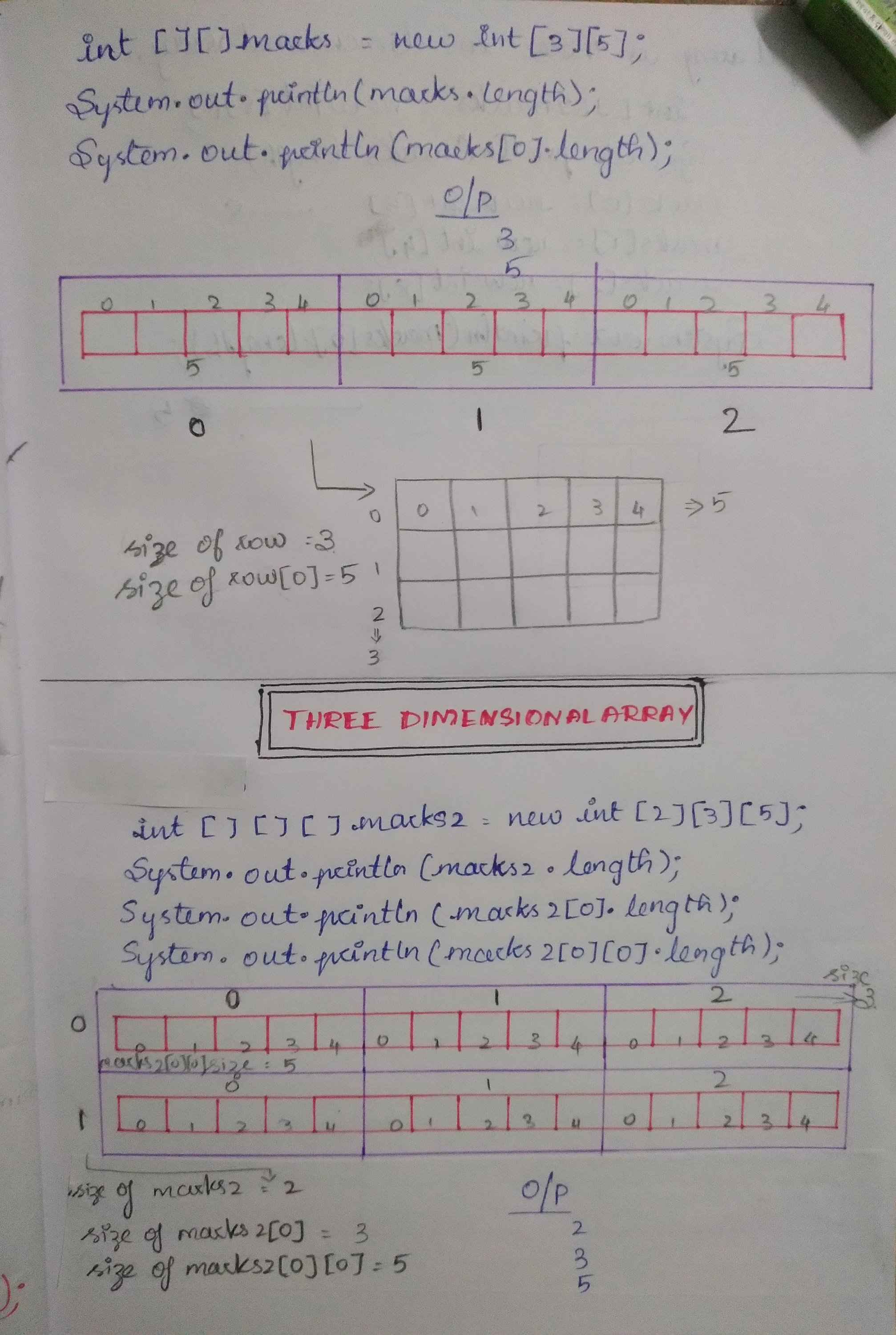
Three Dimensional Array:
- Code snippet for 3D array.
- Data_Type[][][] Name = new int[Tables][Row_Size][Column_Size];
- Tables: Total number of tables it can accept. 2D Array is always a single table with rows and columns. Multi Dimensional array in Java is more than one table with rows and columns.
- Row_Size: Number of Row elements. For example, Row_Size = 5, then the 3D array holds five rows.
- Column_Size: Column elements it can store. Column_Size = 6, then the 3D array holds 6 Columns.
Initialization of Multi Dimensional Array in Java
First Approach
- Initializing elements in a traditional way.
int[][][] Student_Marks = new int[3][5][4];
Second Approach
int[][][] EmployeeArr = { { {10, 20, 30}, {50, 60, 70}, {80, 90, 100}, {110, 120, 130} }, { {15, 25, 35}, {22, 44, 66}, {33, 55, 77}, {78, 57, 76} } };
Here, We did not mention the data level, row size, and column size. The compiler will calculate the size by checking the number of elements inside the row and column.
REFERENCES:
https://www.tutorialgateway.org/multi-dimensional-array-in-java/ https://www.tutorialgateway.org/two-dimensional-array-in-java/ https://www.scientecheasy.com/2021/08/multidimensional-array-in-java.html/ https://www.w3schools.com/java/java_arrays_multi.asp https://www.geeksforgeeks.org/multidimensional-arrays-in-java/ https://www.programiz.com/java-programming/multidimensional-array
Leave a comment